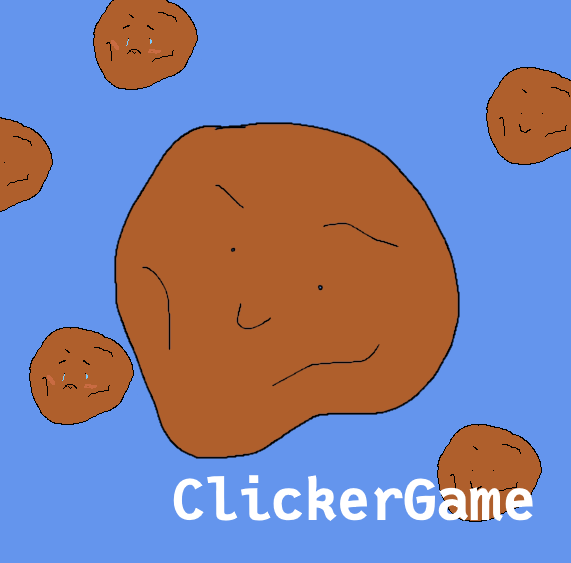
This is a game I made in 2015 for a friend who kept cheating in Cookie Clicker by using auto clickers.
This game is made in C# and the MonoGame framework.
Gameplay
The point of this game is to click the cookie and gain cookies. These cookies can be used to purchase buildings which will increase your cookie amount over time.
Code
For this project I created a Button UI class which I have used in a few other MonoGame project, such as Duck - The Rip Offering.
Button Class
class Button
{
#region Fields
//Text
public string Text { get; set; }
public SpriteFont Font { get; set; }
public Color FontColor { get; set; }
public int FontSize { get; set; }
//Image
public Texture2D SourceTexture { get; set; }
private Texture2D HoverTexture { get; set; }
private Texture2D ClickTexture { get; set; }
public string Tooltip { get; set; }
//Misc
public Rectangle Position { get; set; }
public Vector2 VectorPosition { get; set; }
//Drawing
private bool DrawText = false;
private bool DrawSourceImage = false;
private bool DrawHoverTexture = false;
private bool DrawClickTexture = false;
private bool DrawTooltip = false;
public float DelayToTooltip;
public bool Visible = true;
public bool Enabled = true;
#endregion
#region Constructors
#region Text
public Button(Rectangle position, string text, SpriteFont font)
{
Text = text;
Font = font;
Position = position;
DrawText = true;
}
public Button(Rectangle position, string text, SpriteFont font, Color fontColor, int fontSize)
{
Font = font;
FontColor = fontColor;
FontSize = fontSize;
Text = text;
Position = position;
DrawText = true;
}
#endregion
#region Graphics
public Button(Rectangle position, Texture2D sourceTexture)
{
Position = position;
SourceTexture = sourceTexture;
VectorPosition = new Vector2(position.X, position.Y);
DrawSourceImage = true;
}
public Button(Rectangle position, Texture2D sourceTexture, Texture2D hoverTexture)
{
Position = position;
SourceTexture = sourceTexture;
HoverTexture = hoverTexture;
DrawSourceImage = true;
}
public Button(Rectangle position, Texture2D sourceTexture, Texture2D hoverTexture, string toolTip, SpriteFont font, Color fontColor)
{
Position = position;
SourceTexture = sourceTexture;
HoverTexture = hoverTexture;
Tooltip = toolTip;
Font = font;
FontColor = fontColor;
DrawSourceImage = true;
}
public Button(Rectangle position, Texture2D sourceTexture, Texture2D hoverTexture, Texture2D clickTexture)
{
Position = position;
SourceTexture = sourceTexture;
HoverTexture = hoverTexture;
ClickTexture = clickTexture;
DrawSourceImage = true;
}
public Button(Rectangle position, Texture2D sourceTexture, Texture2D hoverTexture, Texture2D clickTexture, string toolTip, SpriteFont font, Color fontColor)
{
Position = position;
SourceTexture = sourceTexture;
HoverTexture = hoverTexture;
ClickTexture = clickTexture;
Tooltip = toolTip;
Font = font;
FontColor = fontColor;
DrawSourceImage = true;
}
#endregion
#endregion
public void Draw(SpriteBatch batch)
{
if (Visible)
{
if (DrawTooltip)
{
batch.DrawString(Font, Tooltip, new Vector2(Position.X, Position.Y), FontColor);
}
if (DrawClickTexture)
{
var Pos = new Rectangle(Position.X, Position.Y, ClickTexture.Width, ClickTexture.Height);
batch.Draw(ClickTexture, Pos, Color.White);
}
else if (DrawHoverTexture)
{
var Pos = new Rectangle(Position.X, Position.Y, HoverTexture.Width, HoverTexture.Height);
batch.Draw(HoverTexture, Pos, Color.White);
}
else if (DrawSourceImage)
{
batch.Draw(SourceTexture, Position, Color.White);
}
if (DrawText)
{
batch.DrawString(Font, Text, new Vector2(Position.X, Position.Y), FontColor);
}
}
}
public void Update(MouseState state)
{
IsHovering(state);
IsClicked(state);
IsReleased(state);
}
public bool IsHovering(MouseState state)
{
var mousePos = new Point(state.X, state.Y);
if (Position.Contains(mousePos))
{
if (HoverTexture != null)
DrawHoverTexture = true;
if (!string.IsNullOrEmpty(Tooltip))
{
DrawTooltip = true;
}
return true;
}
DrawClickTexture = false;
DrawHoverTexture = false;
return false;
}
public bool IsClicked(MouseState state)
{
if (IsHovering(state))
{
if (state.LeftButton == ButtonState.Pressed)
{
if (ClickTexture != null)
DrawClickTexture = true;
return true;
}
}
DrawClickTexture = false;
return false;
}
public bool IsReleased(MouseState state)
{
if (IsHovering(state))
{
if (state.LeftButton == ButtonState.Released)
{
DrawClickTexture = false;
return true;
}
}
return false;
}
}
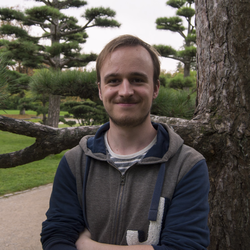